내가 하고싶은 것 :
1.text에 내 위치를 입력하고 내 위치 가져오기 버튼 누르면 DB에 저장된 데이터들의 x, y 좌표 값들을 이용해서 거리 계산후 DB에 저장 및 거리 순 정렬
2. 근처 wifi 정보보기 누르면 거리 짧은 순 20개 화면에 띄워주기
버튼을 눌렀을 때 동작하는 건 javascript로 작성
방법이 3가지가 있는데 addEventListener 사용하는게 권장되는 방식이라고 함.
<button id="my-Space">내 위치 가져오기</button>
<script>
const mySpace = document.getElementById("my-Space");
mySpace.addEventListener('click', () => {
document.body.style.backgroundColor = 'red';
});
</script>
이거는 버튼을 눌렀을 때 배경색을 바꿔주는 함수이고 나는 여기에 입력한 x, y좌표를 보내서 거리를 계산하는 함수를 호출해줄 거임.
흠 상당히 까다로워
<div>
LAT: <input type="text" id="x" value="0.0">,
LNT: <input type="text" id="y" value="0.0">
<button id="my-Space" onclick="calDist_()">내 위치 가져오기</button>
<script>
function calDist_() {
var x = document.getElementById('x').value;
var y = document.getElementById('y').value;
api.APIService.calDist(x,y)
}
</script>
<input type="button" value="근처 WIPI 정보 보기">
</div>
일단 이렇게 만들어줬고 <script>문 안에서 자바 스크립트 코드를 씀.
근데 저기서 console.log해서 input으로 입력된 값 뽑으려 했는데 절대 안 나옴 ..왜지 ?
그리고 여기서 이제 java 함수 호출을 해줘야하는데 ajax를 이용해야한다고 한다.
- 기본개념
https://jae-jae.tistory.com/504
[AJAX] AJAX 정의, 함수의 사용 형식, 주요 매개변수 옵션, 예제 코드
AJAX 정의 Ajax는 Asynchronous(비동기) JavaScript and XML의 약어로 비동기로 데이터 통신을 처리하기 위한 기술로 특정 언어나 플랫폼 혹은 프레임워크나 라이브러리를 뜻하는 것이 아닌 자바스크립트로
jae-jae.tistory.com
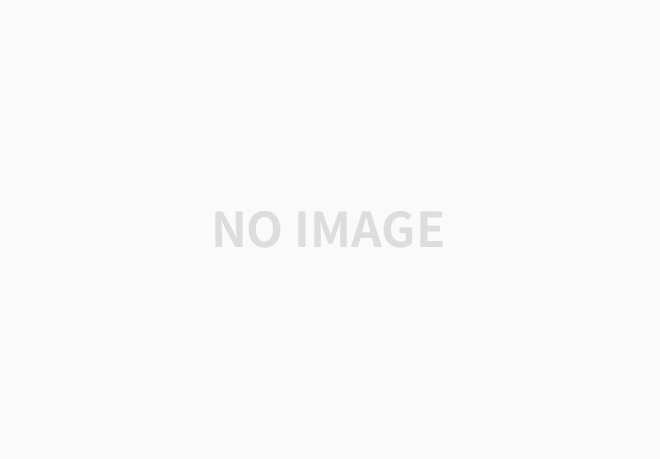
어노테이션을 쓰려고 하니까 안 된다 ㅠ
https://sunny-jang.tistory.com/35
이클립스 Lombok @Getter,@Setter Annotation 인식 안됨 [해결]
Lombok이라는 라이브러리는 클래스 상단에 어노테이션을 입력해 기본적으로 생성되야 하는 메서드들을 생성해주는 것을 배워서 사용해 보려는데 jar파일도 다운 받아서 라이브러리에 넣어주고 @G
sunny-jang.tistory.com
여기 참고해서도 해봤는데 안 된다.
흠.. 어떡하지 ? 난 스프링으로 만든게 아니어서 그런가
찾아보면 import 문이 다 org.springframework. ~~..이런식으로 되어있음.
그냥 자바스크립트에서 어떻게든 해보기로함
<index.jsp>
<div>
LAT: <input type="text" id="x" value="0.0">,
LNT: <input type="text" id="y" value="0.0">
<button id="my-Space" onclick="calDist_()">내 위치 가져오기</button>
<script>
<% double xx = 0; double yy = 0; %>
function calDist_() {
var x = document.getElementById('x').value;
var y = document.getElementById('y').value;
<%=xx %> = x;
<%=yy %> = y;
<% apiService.calDist(xx, yy); %>
}
</script>
<input type="button" value="근처 WIPI 정보 보기">
</div>
<APIService.java>
public void calDist(double x, double y) {
System.out.println("실행은 되니 ?");
try {
Class.forName("org.sqlite.JDBC");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet rs = null;
String url_value = "jdbc:sqlite:C:\\dev_web\\sqlite-tools-win32-x86-3430000\\wifi.db";
try {
connection = DriverManager.getConnection(url_value);
String sql = "select x, y from wifi_list";;
preparedStatement = connection.prepareStatement(sql);
rs = preparedStatement.executeQuery();
while(rs.next()) {
double x_value = rs.getDouble("x");
double y_value = rs.getDouble("y");
double dist = distance(x, y, x_value, y_value);
String updatesql = "update wifi_list set dist= ?";
preparedStatement = connection.prepareStatement(updatesql);
preparedStatement.setDouble(1, dist);
preparedStatement.executeUpdate();
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null && !rs.isClosed()) {
rs.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if(preparedStatement != null && !preparedStatement.isClosed()) {
preparedStatement.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if(connection != null && !connection.isClosed()) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public double distance(double lat1,double lon1,double lat2,double lon2) {
double R = 6371; // 지구 반지름 (단위: km)
double dLat = deg2rad(lat2 - lat1);
double dLon = deg2rad(lon2 - lon1);
double a = Math.sin(dLat/2) * Math.sin(dLat/2) +
Math.cos(deg2rad(lat1)) * Math.cos(deg2rad(lat2)) *
Math.sin(dLon/2) * Math.sin(dLon/2);
double c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1-a));
double distance = R * c; // 두 지점 간의 거리 (단위: km)
return distance;
}
public double deg2rad(double deg) {
return deg * (Math.PI/180);
}
이렇게 하고 실행을 해줬더니 입력도 안 했고 클릭도 안 했는데 서버가 엄청 느리게 로딩되면서 데이터베이스에 dist 속성 레코드가 업데이트 되고있음 !!!
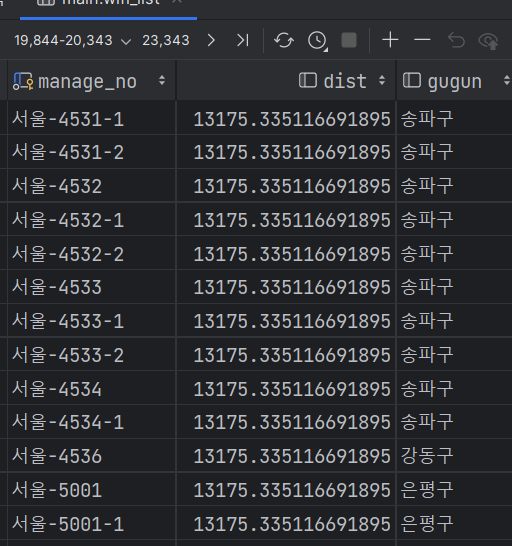
jsp를 실행시키면 자바코드 > dom > 자바스크립트 순으로 실행이 된다더니 자바코드가 먼저 실행되어서 그런가보다 ...
일단 계산은 하나도 안 되고 있음을 알 수 있음.
Controller로 서블릿 매핑 해주고 ajax 이용해서 값 보내는 거 해결했다 !
근데 인제 서버에서 쏴준 값을 jsp에서 받아서 다시 다른 서버로 보내는데
서버에서 request.setAttribute("bm",bm);으로 세팅해서
<table>
<P>북마크를 삭제하시겠습니까?</P>
<thead>
<tr>
<th>북마크 이름</th><td>${bookMark.bookmarkName }</td>
</tr>
<tr>
<th>와이파이명</th><td>${bookMark.wifiName }</td>
</tr>
<tr>
<th>등록일자</th><td>${bookMark.makeDate }</td>
</tr>
</thead>
<tbody>
<tr>
<td><a href="/bookmark/bookmarkList">돌아가기</a> | <button value="" onclick="listdel_(${bookMark.id });">삭제</button></td>
</tr>
</tbody>
</table>
<script>
function listdel_(id) {
location.href="/bookmark/bookmarkdelset?id="+id;
}
</script>
</body>
삭제 버튼에 onclick 일어나면 저 location.href를 그대로 넣어줬는데 안 됐다.
그래서 방법을 찾다가 onclick 일어나면 함수 호출을 하고 서버로 넘겨줄 id값을 함수 인자값으로 넘겨줘서
<script>의 함수에서 id 값을 넘겨주면 된다 !
https://webnamubada.tistory.com/315
'ZB 백엔드 스쿨 > 과제' 카테고리의 다른 글
위, 경도 상의 거리 구하기 (2) | 2023.09.05 |
---|---|
SQLite 설치하고 DataGrip에 연결 (테이블 생성 완료) (0) | 2023.09.02 |
서울시 공공와이파이 정보 OPEN API 받아오기 - (파싱까지 완료) (0) | 2023.09.02 |
Mission1 깜짝과제 03.html 페이징 처리 (0) | 2023.08.12 |
Mission1 깜짝과제 02.조건에 맞는 프로그램 작성하기 (0) | 2023.08.12 |